patatap 动效 moon 分析
前言
刷到一个很 🐂 的网站patatap,好奇它的实现原理,看了下源码,记录一下分析结果。
用到的库
two.js:二维绘图
tween.js:补间引擎
核心就是利用 two.js 绘制图形,然后利用 tween.js 加一些补间动画,过渡。
准备工作
使用 rollup 进行代码打包操作
rollup.config.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| import resolve from "@rollup/plugin-node-resolve"; import commonjs from "@rollup/plugin-commonjs"; import typescript from "@rollup/plugin-typescript";
export default { input: "src/index.ts", output: { file: "bundle.js", }, plugins: [ resolve(), commonjs(), typescript(), ], };
|
moon 动效
代码
common.js(two.js 实例、container 容器等公用内容)
1 2 3 4 5 6 7 8 9 10
| import Two from "two.js";
export const two = new Two({ type: Two.Types.canvas, fullscreen: true, });
export const container: HTMLElement = document.querySelector("#container");
export const center = new Two.Vector(two.width / 2, two.height / 2);
|
index.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129
| import Two from "two.js"; import TWEEN, { Tween } from "@tweenjs/tween.js"; import { Vector } from "two.js/src/vector"; import { center, container, two } from "./common.js";
two.appendTo(container);
const duration = 1000;
let minDimension = Math.min(two.width, two.height);
two.bind("resize", () => { center.x = two.width / 2; center.y = two.height / 2;
minDimension = Math.min(two.width, two.height); });
function makeMoon() { const amount = 64; const half = amount / 2; const destinations: Vector[] = [];
const points = [...Array(amount).keys()].map(() => { destinations.push(new Two.Vector()); return new Two.Anchor(); });
const moon = two.makePath(points); const options = { in: 0, out: 0, };
moon.fill = "#e34f0c"; moon.noStroke();
function resize() { moon.translation.copy(center); } resize();
let animate_in: Tween<typeof options>; let animate_out: Tween<typeof options>; function start() { moon.visible = true; animate_in.start(); }
function onComplete() { animate_out.start(); }
function reset() { if (animate_in) { animate_in.stop(); } if (animate_out) { animate_out.stop(); }
options.in = 0; options.out = 0;
moon.visible = false; moon.rotation = Math.random() * Math.PI * 2;
const radius = minDimension * 0.33;
moon.vertices.map((v, i) => { const pct = i / (amount - 1); const theta = pct * Math.PI * 2;
const x = radius * Math.cos(theta); const y = radius * Math.sin(theta);
destinations[i].set(x, y); if (i < half) { destinations[i].y *= -1; } v.set(x, Math.abs(y)); });
animate_in = new TWEEN.Tween(options) .to({ in: 1 }, duration * 0.5) .easing(TWEEN.Easing.Sinusoidal.Out) .onUpdate(() => { for (let i = half; i < amount; i++) { points[i].lerp(destinations[i], options.in); } }) .onComplete(onComplete);
animate_out = new TWEEN.Tween(options) .to({ out: 1 }, duration * 0.5) .easing(TWEEN.Easing.Sinusoidal.Out) .onUpdate(() => { for (let i = 0; i < half; i++) { points[i].lerp(destinations[i], options.out); } }) .onComplete(reset); }
document.addEventListener("click", () => { reset(); start(); });
two .bind("update", () => { TWEEN.update(); }) .play(); }
makeMoon();
|
分析
- 实例化 two.js,调用 appendTo 跟 dom 元素关联起来
- 构建点集 points,并且使用 destinations 跟 points 建立关联(用于后续构建补间动效)
two.makePath(points)
。创建路径,此时是空路径。
- 设置中心点。利用
moon.translation.copy(center)
让绘制的图形移动到 center 的位置
- 因为中心点有可能会变,实际上在最开始有个绑定
resize
监听时间的逻辑
1 2 3 4 5 6 7
| two.bind("resize", () => { center.x = two.width / 2; center.y = two.height / 2;
minDimension = Math.min(two.width, two.height); });
|
- 核心逻辑:
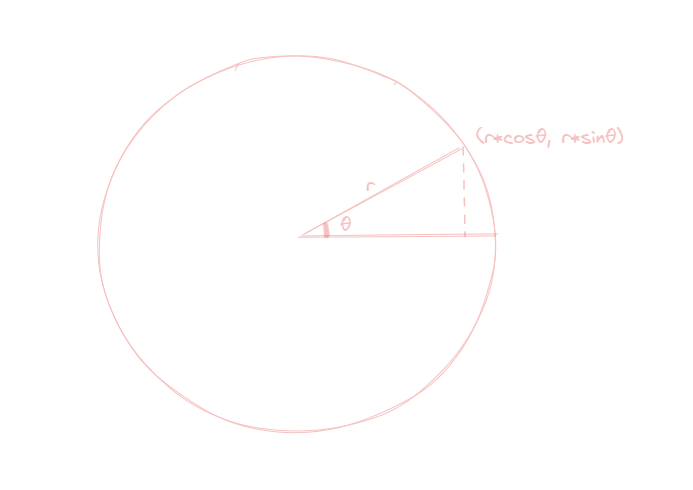
6.3 利用 tween.js 实现补间动效
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| const options = { in: 0, out: 0, }; animate_in = new TWEEN.Tween(options) .to({ in: 1 }, duration * 0.5) .easing(TWEEN.Easing.Sinusoidal.Out) .onUpdate(() => { for (let i = half; i < amount; i++) { points[i].lerp(destinations[i], options.in); } }) .onComplete(onComplete);
|
1 2 3 4 5 6 7 8 9 10
| animate_out = new TWEEN.Tween(options) .to({ out: 1 }, duration * 0.5) .easing(TWEEN.Easing.Sinusoidal.Out) .onUpdate(() => { for (let i = 0; i < half; i++) { points[i].lerp(destinations[i], options.out); } }) .onComplete(reset); }
|
animate_in 对后一半的点进行处理,这样子前一半在 y 轴上面,后一半在 y 轴下面,就会形成一个圆。而 animate_out 对前一半的点进行处理,让它们过渡到 y 轴下面,这样子会只有重叠成一个 y 轴下面的半圆。动效效果就是圆折成半圆,完成的时候,隐藏路径。
注意点
开启 two.js 的动画时,还需要绑定update
事件,当update
事件触发时,调用TWEEN.update()
更新TWEEN
。
1 2 3 4 5
| two .bind("update", () => { TWEEN.update(); }) .play();
|
效果
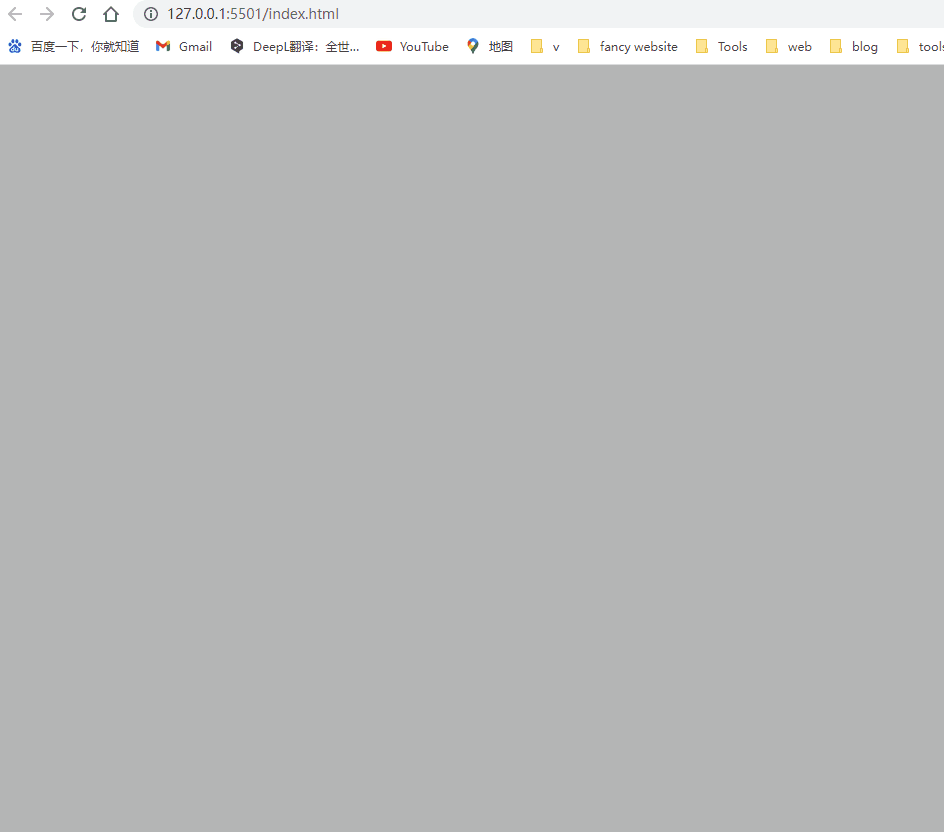