调试用到的几种断点
VSCode
1. 条件断点
顾名思义,就是只有满足条件才会中断的断点。
1.1 表达式断点
在表达式结果为真时中断。
简单使用:
1 2 3 4 5 6
| function add(a, b) { return a + b; }
add(1, 2); add(2, 3);
|
首先,添加普通的断点。
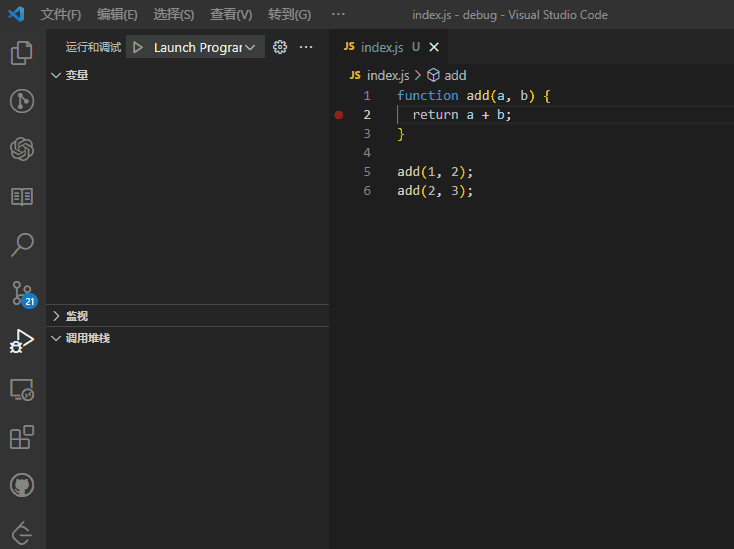
可以看到会断两次,但是如果添加的是条件断点的话,就可能不是断两次了。
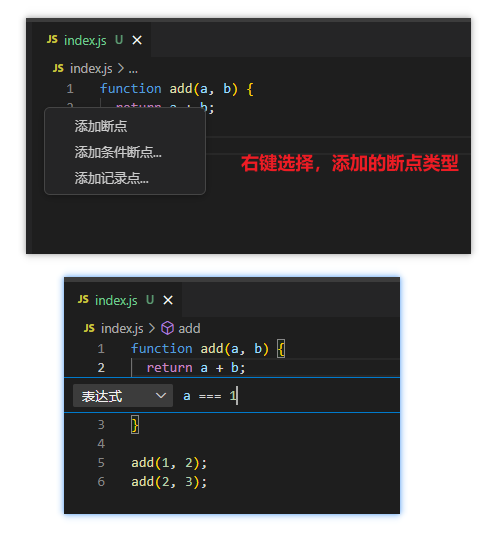
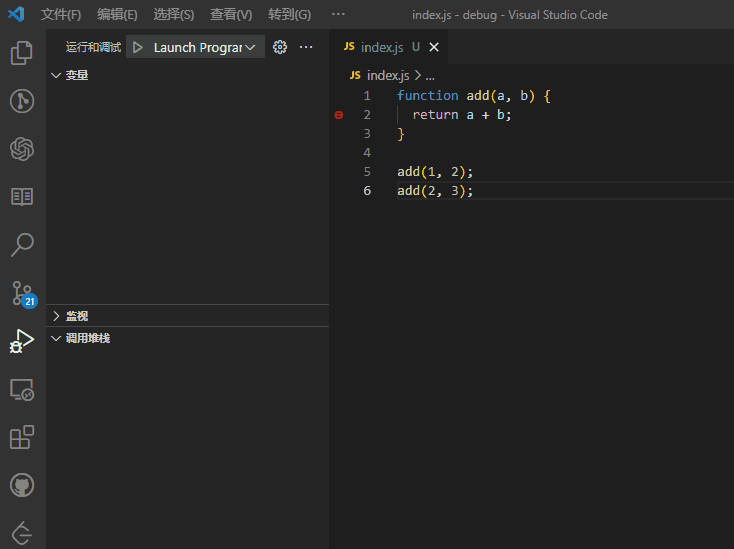
另外,VSCode的断点是即添(改)即用的,所以配合条件断点能干很多事情:
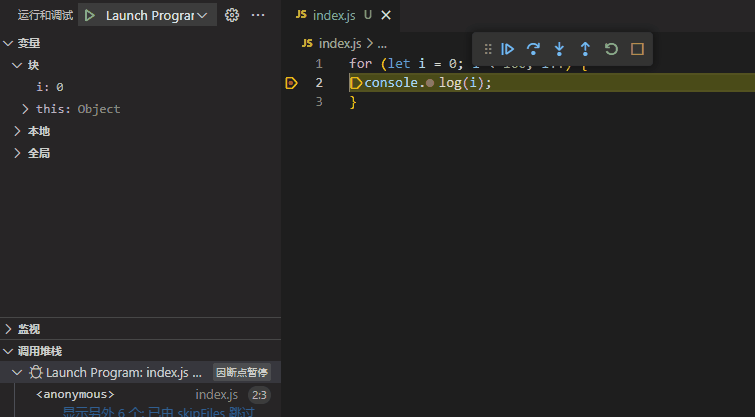
1.2 命中次数中断
当命中次数满足条件才会中断。
\color{red}{不能只是输入一个数字,而应是== 9
或> 9
这种形式}
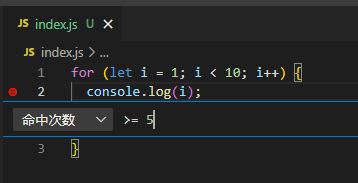
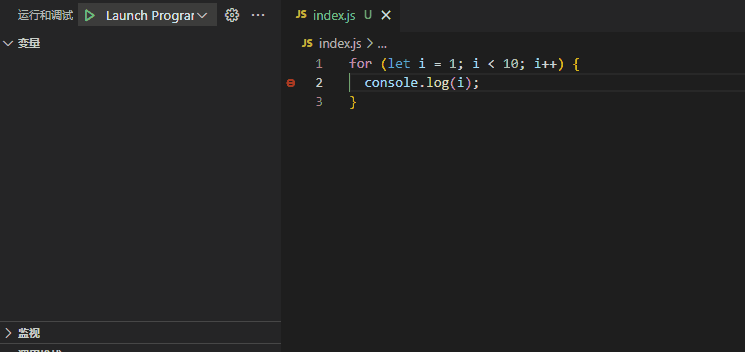
2. 记录点
断点命中时记录的信息。直接输入的内容会当成字符串来处理,要输入表达式的话,需要用{}
包住。
\color{red}{条件节点和记录点不能混合使用,混合使用,记录点会失效。}
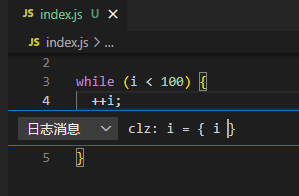
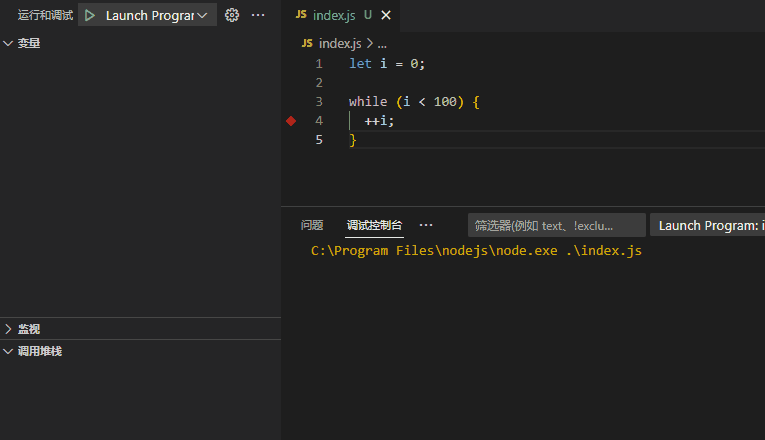
实际上,记录点和console
效果基本一样。不过,记录点并不会污染代码。
3. 异常断点
出现异常后才会中断的断点。会分为捕获和未捕获两种。
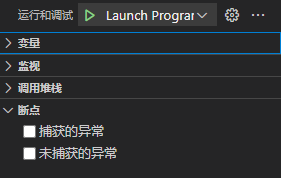
异常断点的好处自然就是能够知道出现异常时的一些变量信息、调用堆栈信息。
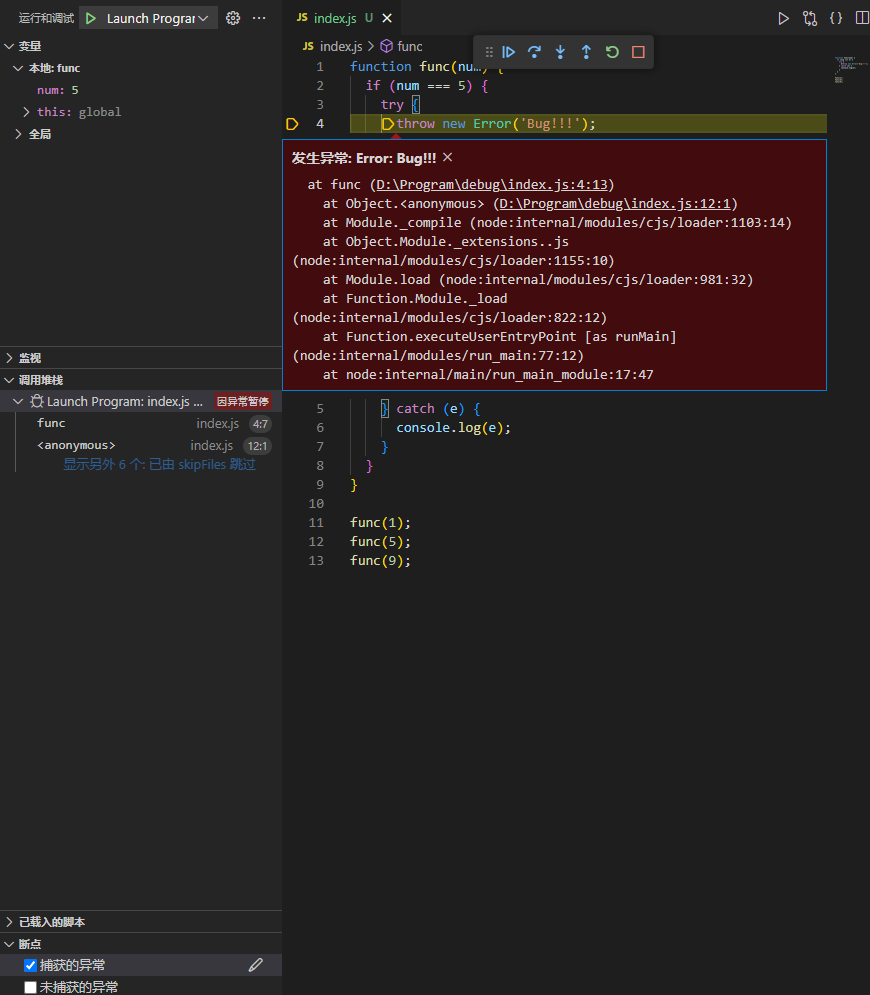
4.内联断点
只有当执行到与内联断点关联的行时,才会命中内联断点。(不知道为什么网上都说是列)
把光标移动到要断的位置,然后点击Shift + F9
。或者点击运行>新建断点
。
内联断点比较适合调试一行中包含多个语句的代码,比如for循环,可以等到满足条件时,再进入循环体。这时候,调试自由度比条件断点要高一点点。
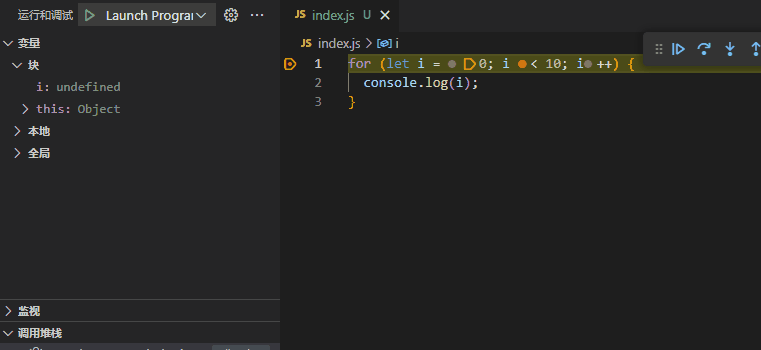
Chrome
这部分介绍的是Chrome提供的一些断点。但是,也是可以通过VSCode去调试的,只不过需要在Chrome中设置断点。(下面为了方便录屏就不用VSCode来调试了)
1. 事件断点
添加事件断点后,当触发该事件时,就会中断。可以用于查看一下组件库触发事件后会进行哪些操作。
1 2 3 4 5 6 7 8 9 10
| <button onclick="handleClick()">click</button>
<script> function handleClick() { let a = 3; let b = 4;
console.log(a + b); } </script>
|
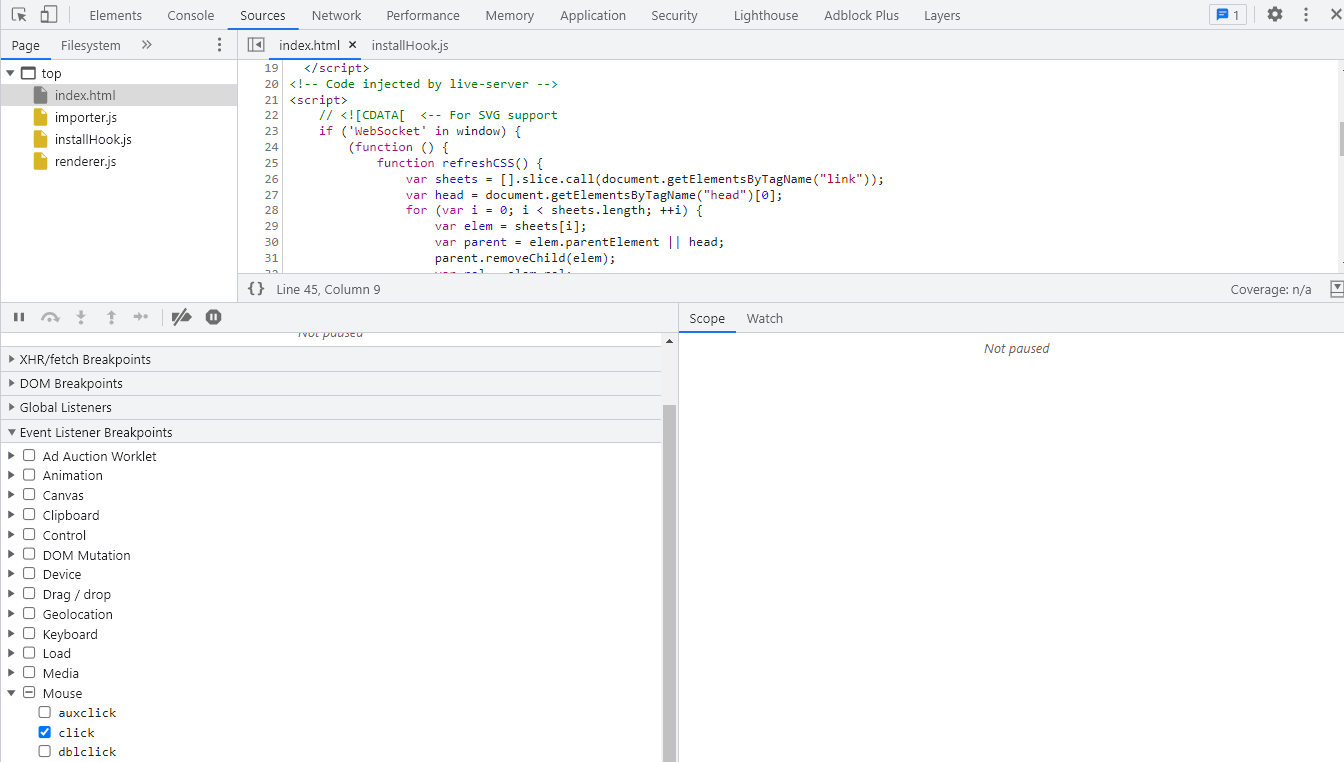
2. DOM断点
DOM断点的设置并不是在Sources面板中,而是在Elements面板中选中DOM元素,右键,选择Break on
设置,一共有三种类型。
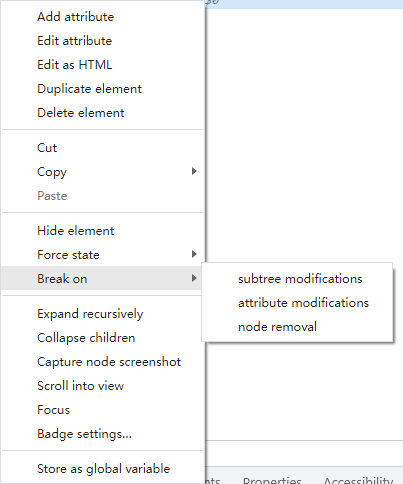
2.1 subtree modifications
(子树修改)
当前选择的节点的子节点被移除或添加,以及子节点的内容(不包括属性)更改时触发。
HTML:
1 2 3 4 5 6 7
| <div class="father"> <div class="son">son</div> </div>
<button onclick="handleRemove()">remove</button> <button onclick="handleAdd()">add</button> <button onclick="handleChange()">change</button>
|
CSS:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <style> .father { display: flex; justify-content: center; align-items: center; width: 200px; height: 200px; background-color: pink; }
.son { width: 100px; height: 100px; background-color: purple; line-height: 100px; text-align: center; color: #fff; } </style>
|
JavaScript:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <script> const father = document.querySelector('.father'); const son = document.querySelector('.son');
function handleRemove() { father.removeChild(son); }
function handleAdd() { father.appendChild(son); }
function handleChange() { son.textContent = 'ccc'; } </script>
|
首先,设置好断点。
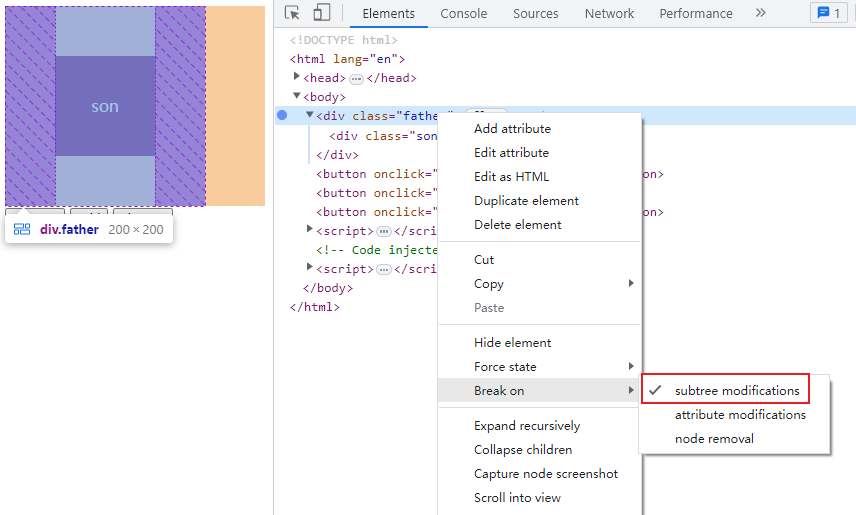
接着,点击三个按钮的其中一个都会中断。
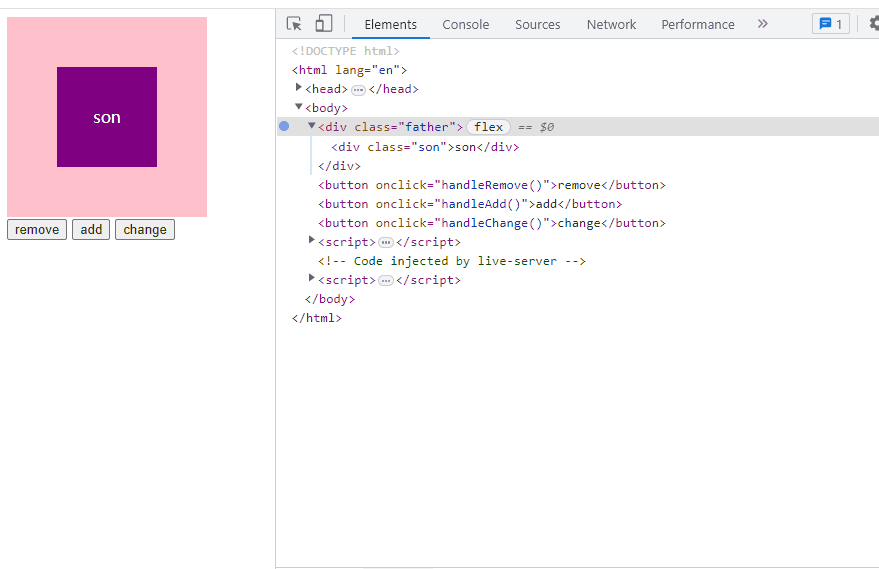
2.2 attribute modifications
(属性修改)
当前节点添加、删除、更改属性值时触发。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title>
<style> div { width: 100px; height: 100px; text-align: center; line-height: 100px; }
.bg { background-color: pink; } </style> </head> <body> <div data-name="clz"> clz </div>
<button onclick="handleAdd()">add</button> <button onclick="handleRemove()">remove</button> <button onclick="handleChange()">change</button>
<script> const div = document.querySelector('div');
function handleAdd() { div.classList.add('bg'); }
function handleRemove() { div.classList.remove('bg'); }
function handleChange() { div.setAttribute('data-name', 'czh'); } </script> </body> </html>
|
和子树修改基本一样操作。
2.3 node removal
(节点移除)
当前节点被移除时触发。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title>
<style> div { width: 100px; height: 100px; text-align: center; line-height: 100px; } </style> </head> <body> <div> clz </div> <button onclick="handleRemove()">remove</button>
<script> const div = document.querySelector('div');
function handleRemove() { div.parentElement.removeChild(div); } </script> </body> </html>
|
顺带一提:DOM断点能同时添加多种类型。
3. 请求断点
当发送请求的时候中断。如果不输入内容则是所有请求都中断,如果输入内容,则是当url
中包含该内容的请求会中断。
请求断点不会考虑请求能不能发送到服务器。而是在发送请求的时候中断。
1 2 3 4 5 6 7 8 9 10 11
| fetch("http://localhost:8088/getInfo") .then((res) => { console.log(res); });
fetch('http://localhost:8088/postInfo', { method: 'POST' }) .then((res) => { console.log(res); })
|
所有请求都中断:
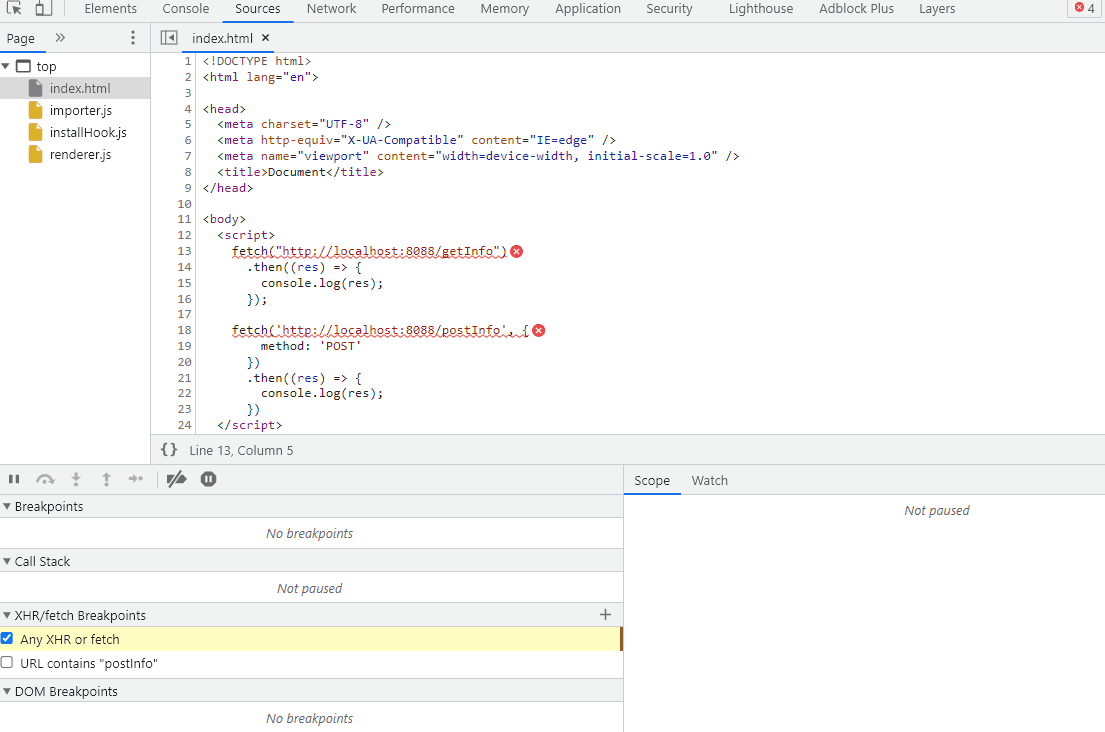
只有url
包含给定内容的请求才会被中断:
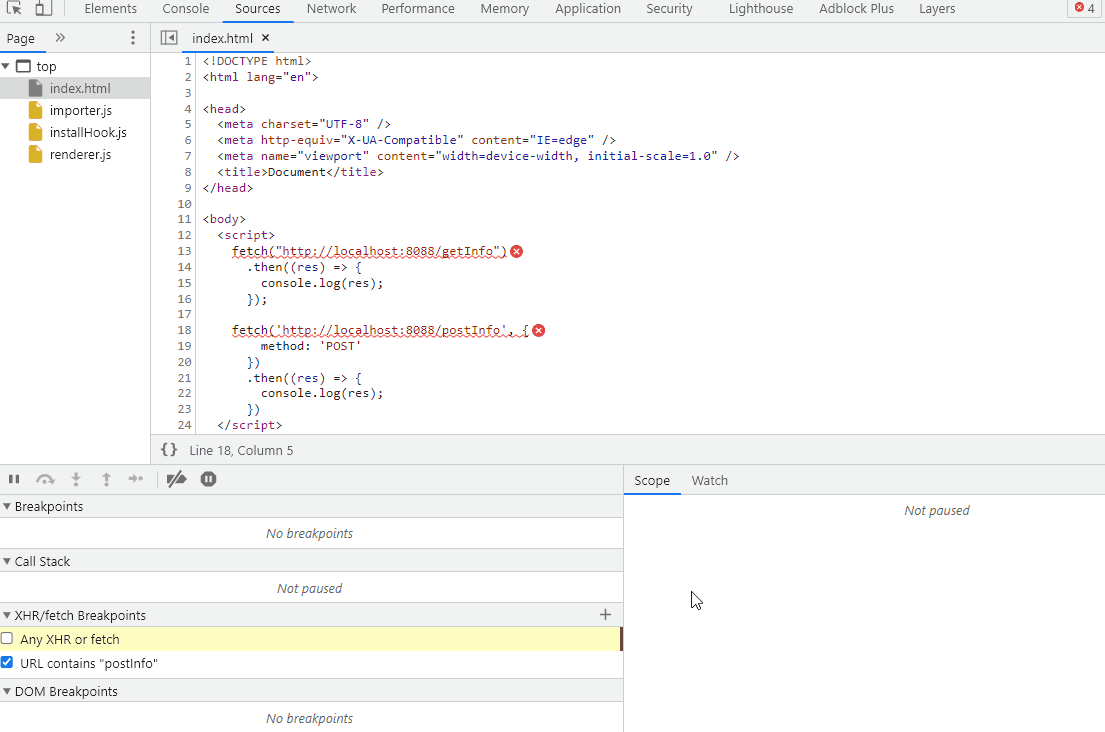